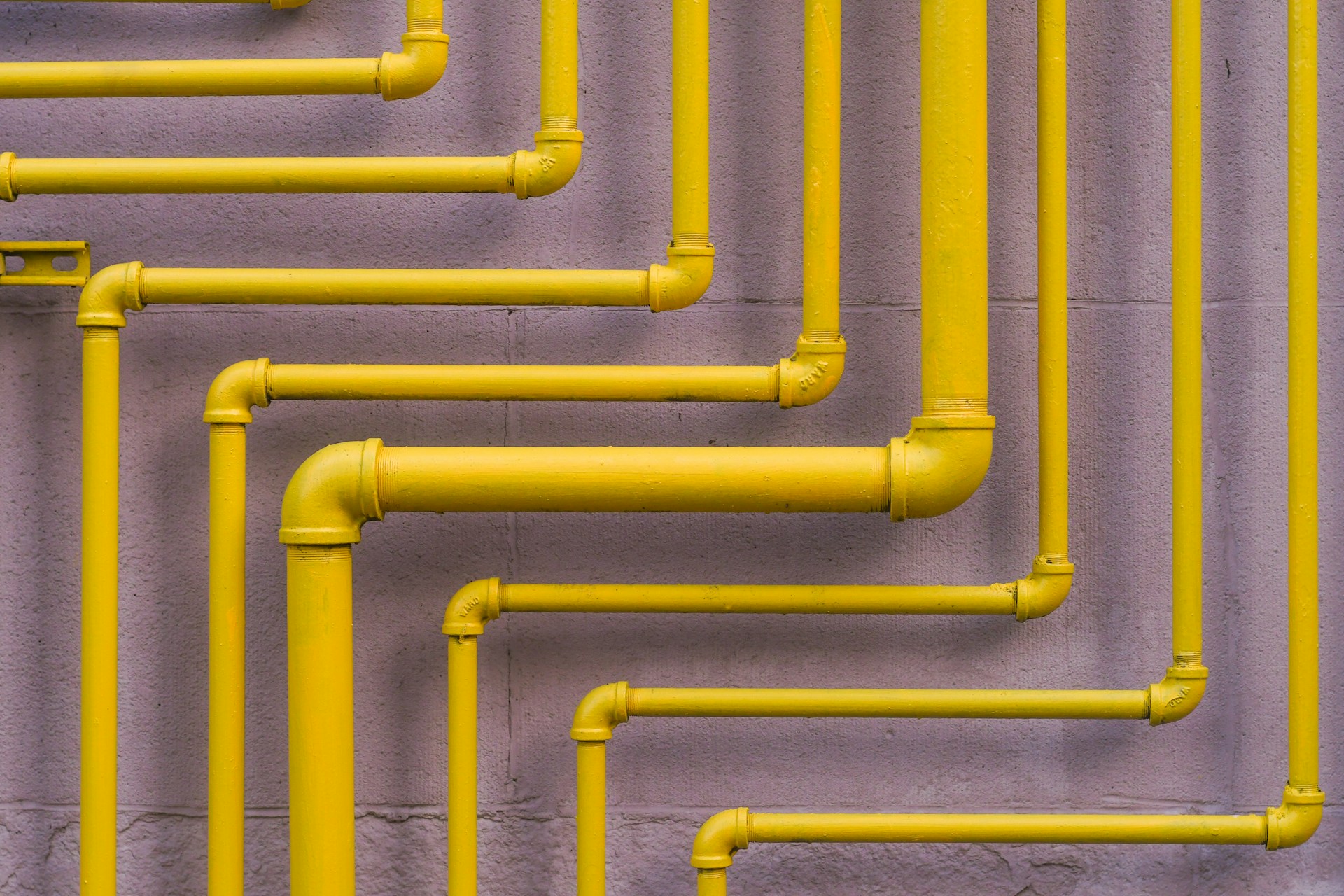
What are Laravel Pipelines?
Published on Share
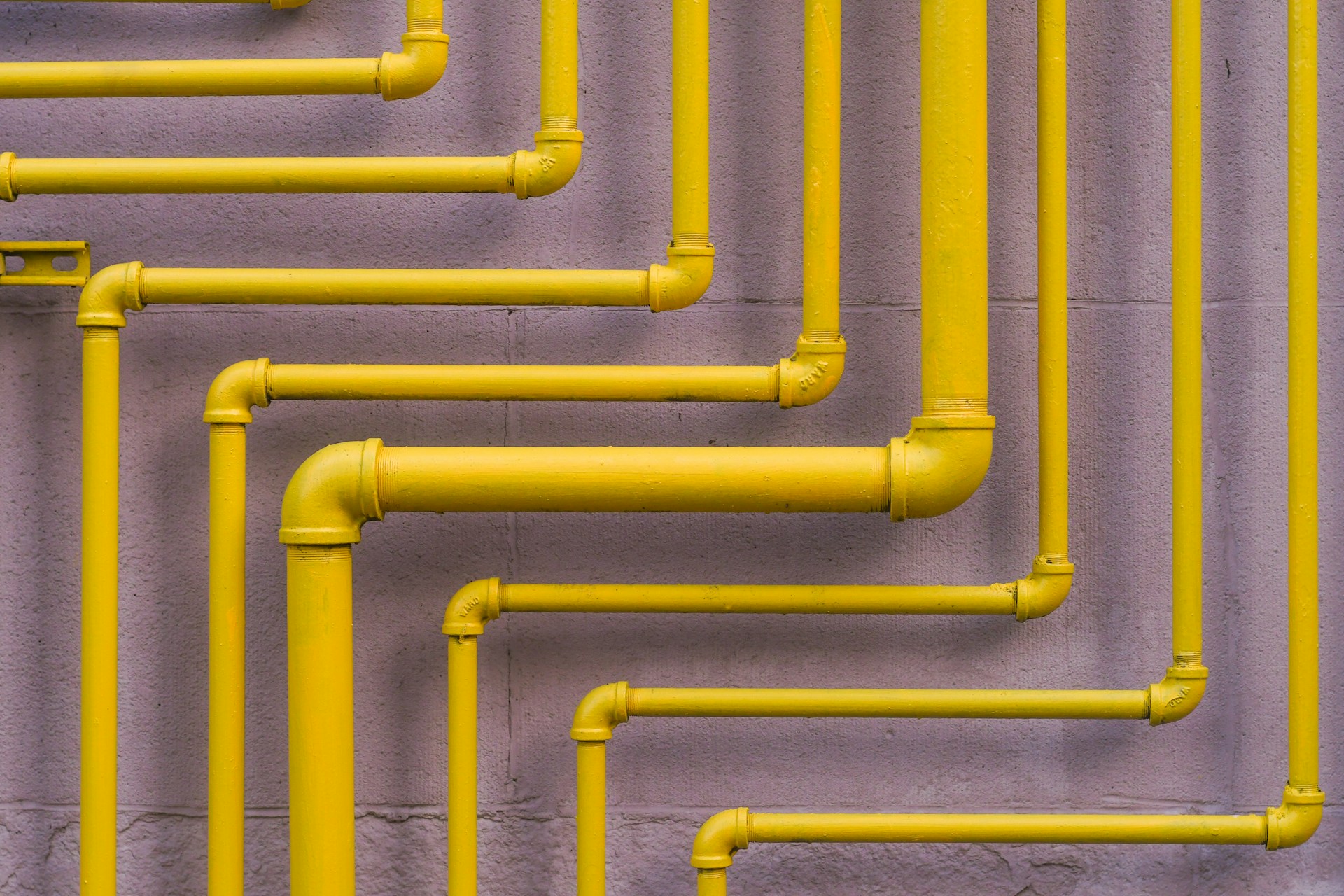
Introduction
In modern web development, efficiently managing and processing data is crucial for building scalable and maintainable applications. Laravel offers a powerful tool for streamlining these tasks: the Laravel Pipeline.
So, what exactly is a Laravel Pipeline?
At its core, a pipeline is a design pattern that allows you to pass data through a series of processing stages, where each stage performs a specific task or transformation. This concept is akin to an assembly line in a factory, where raw materials are progressively refined through a series of steps until they become a finished product.
Laravel Pipelines provide a clean and elegant way to manage such sequential processing tasks within your applications. They allow you to build complex data handling workflows in a modular and maintainable way, making your code more readable and easier to manage.
How Pipelines Are Implemented in Laravel
In Laravel, the Pipeline class is used to implement this pattern. The Pipeline class is designed to handle a sequence of operations that can be applied to a value or data set. Laravel Pipelines make it easy to create and manage complex processing chains by allowing you to define each step of the process as a separate "pipe."
Here’s a basic overview of how Laravel Pipelines work:
-
Pipeline Definition: You define a pipeline by creating an instance of the
Illuminate\Pipeline\Pipeline
class and adding various pipes (steps) to it. -
Pipe Definition: Each pipe is a callable function or class that processes the data in some way. These pipes are executed in the order they are added.
-
Data Flow: Data is passed through the pipeline from the initial value through each pipe in sequence. Each pipe processes the data and then passes it to the next pipe in the chain.
Here is a very simple example to illustrate how a pipeline works in Laravel:
use Illuminate\Pipeline\Pipeline;
$initialData = 'Ich schneide Kopfsalat!';
$result = app(Pipeline::class)
->send($inputData) // Send initial data
->through([
\App\Pipes\FirstPipe::class,
\App\Pipes\SecondPipe::class,
]) // Define the pipes
->thenReturn(); // Execute the pipeline and return the result
In this example:
-
$inputData
is the initial data being processed. -
FirstPipe
andSecondPipe
are classes that define the processing steps. - The
through
method registers these pipes with the pipeline. -
thenReturn
executes the pipeline and returns the processed data.
Why Should I Use Laravel Pipelines?
Laravel Pipelines offer a powerful way to handle sequential data processing tasks, but like any tool, they’re not always the right fit for every situation. Deciding whether to use pipelines in your application requires consideration of several factors, including the complexity of the task at hand and how well pipelines integrate with your existing code style and architecture.
When to Consider Using Laravel Pipelines
-
Complex Data Processing
Pipelines shine when dealing with complex data processing workflows. If you find yourself needing to apply multiple transformations, filters, or steps to your data, using a pipeline can help you manage these processes in a clean, modular fashion. For instance, if you have a multi-step process for handling user registration, where you need to validate input, create a user record, send a confirmation email, and log the event, a pipeline can organize these tasks efficiently.
Example Use Case: Processing a user's profile update, where each step involves different validation, transformation, and storage operations.
-
Separation of Concerns
Pipelines are useful for separating different concerns in your application. By defining each step in a separate pipe, you create a clear separation between different parts of your data processing logic. This modular approach enhances readability and maintainability, making it easier to manage complex workflows.
Example Use Case: Handling a multi-step order processing system where each stage of the process (e.g., validation, inventory check, payment processing) is clearly defined and separated.
-
Reusability and Composability
When you have reusable processing steps that could be applied in different contexts, pipelines offer a way to compose these steps into a cohesive workflow. This reusability can reduce code duplication and make your application more modular.
Example Use Case: Applying a set of common data transformations to different types of input across various parts of your application.
When to Think Twice
While pipelines are powerful, they might not be the best choice for every scenario. Here are some cases where using pipelines might not be necessary:
-
Simple Operations
For simple, straightforward tasks where only a few steps are involved, using pipelines might add unnecessary complexity. If your processing steps are minimal, the overhead of creating and managing a pipeline might outweigh the benefits.
Example: A basic operation like creating a user and immediately dispatching an event might be more straightforward to handle directly within a single method or service.
-
Performance Concerns
In some cases, the overhead introduced by pipelines may impact performance, especially if the pipeline involves many steps or operates on large datasets. Ensure that the use of pipelines aligns with your performance requirements. -
Existing Code Style
Consider whether pipelines fit well with your existing code style and architecture. If your project already has a well-established way of handling data processing that aligns with your current codebase, introducing pipelines might not be necessary and could disrupt your existing structure.
Complex Use Case: Multi-Step Order Processing Pipeline
To illustrate the power and flexibility of Laravel Pipelines, let’s explore a more complex example: a multi-step order processing system. This scenario involves several stages, including validation, inventory checks, payment processing, and order confirmation. Each stage requires distinct operations, and using a pipeline helps to manage these operations in a clear and organized manner.
Scenario
Imagine you are building an e-commerce application where customers place orders for products. Each order goes through a series of processing steps before it is finalized. These steps include:
- Order Validation: Ensure that the order data is valid and complete.
- Inventory Check: Verify that the products are in stock and available.
- Payment Processing: Handle the payment transaction.
- Order Confirmation: Update the order status and send a confirmation email to the customer.
Implementation
Here's how you can implement this using Laravel Pipelines:
Defining the Pipeline Create a pipeline instance to handle the order processing. You’ll define each step of the process as a separate pipe class.
use Illuminate\Pipeline\Pipeline;
use App\Pipes\OrderValidation;
use App\Pipes\InventoryCheck;
use App\Pipes\PaymentProcessing;
use App\Pipes\OrderConfirmation;
$order = app(Pipeline::class)
->send($orderData) // Send the initial order data
->through([
OrderValidation::class,
InventoryCheck::class,
PaymentProcessing::class,
OrderConfirmation::class,
]) // Define the pipes
->thenReturn(); // Execute the pipeline and return the result
The Pipe Classes Each pipe class handles a specific part of the processing. Here’s an overview of what each class might look like:
OrderValidation.php
namespace App\Pipes;
class OrderValidation
{
public function handle($orderData, \Closure $next)
{
// Validate the order data
if (!$this->isValid($orderData)) {
throw new \Exception("Invalid order data");
}
return $next($orderData); // Pass the data to the next pipe
}
protected function isValid($orderData)
{
// Validation logic here
return true;
}
}
InventoryCheck.php
namespace App\Pipes;
class InventoryCheck
{
public function handle($orderData, \Closure $next)
{
// Check inventory levels
if (!$this->isInStock($orderData)) {
throw new \Exception("Products out of stock");
}
return $next($orderData); // Pass the data to the next pipe
}
protected function isInStock($orderData)
{
// Inventory checking logic here
return true;
}
}
PaymentProcessing.php
namespace App\Pipes;
class PaymentProcessing
{
public function handle($orderData, \Closure $next)
{
// Process the payment
if (!$this->processPayment($orderData)) {
throw new \Exception("Payment failed");
}
return $next($orderData); // Pass the data to the next pipe
}
protected function processPayment($orderData)
{
// Payment processing logic here
return true;
}
}
OrderConfirmation
namespace App\Pipes;
class OrderConfirmation
{
public function handle($orderData, \Closure $next)
{
// Confirm the order and send a confirmation email
$this->confirmOrder($orderData);
$this->sendConfirmationEmail($orderData);
return $orderData; // Return the final result
}
protected function confirmOrder($orderData)
{
// Order confirmation logic here
}
protected function sendConfirmationEmail($orderData)
{
// Email sending logic here
}
}
Benefits
By using Laravel Pipelines for this order processing workflow:
- Modularity: Each step is encapsulated in its own class, making the system easy to modify and extend.
- Readability: The pipeline provides a clear sequence of operations, improving code readability.
- Maintainability: Changes to one part of the process (e.g., payment processing) can be made without affecting other steps.
Thats it.
From the blog
Random posts from my blog.
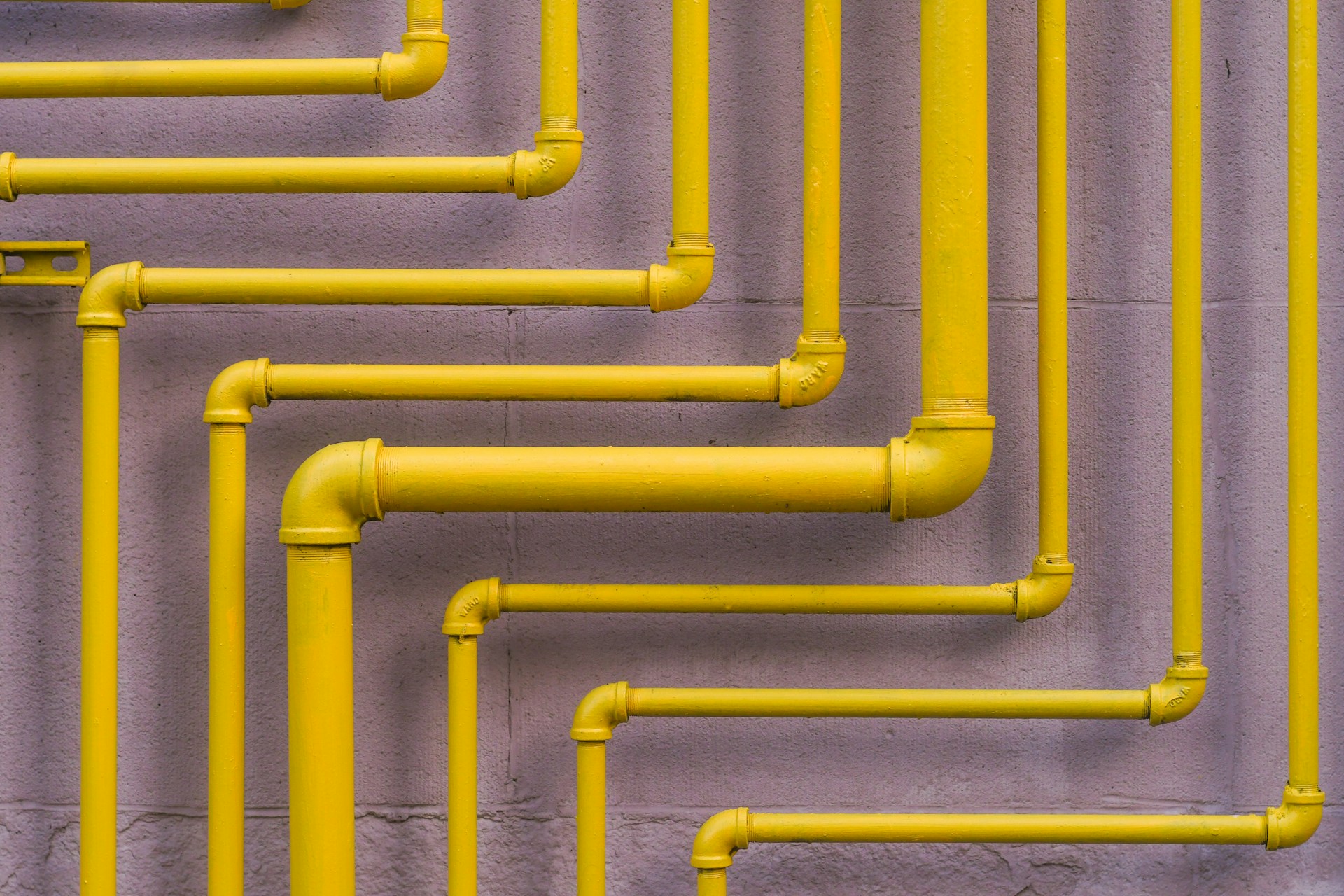
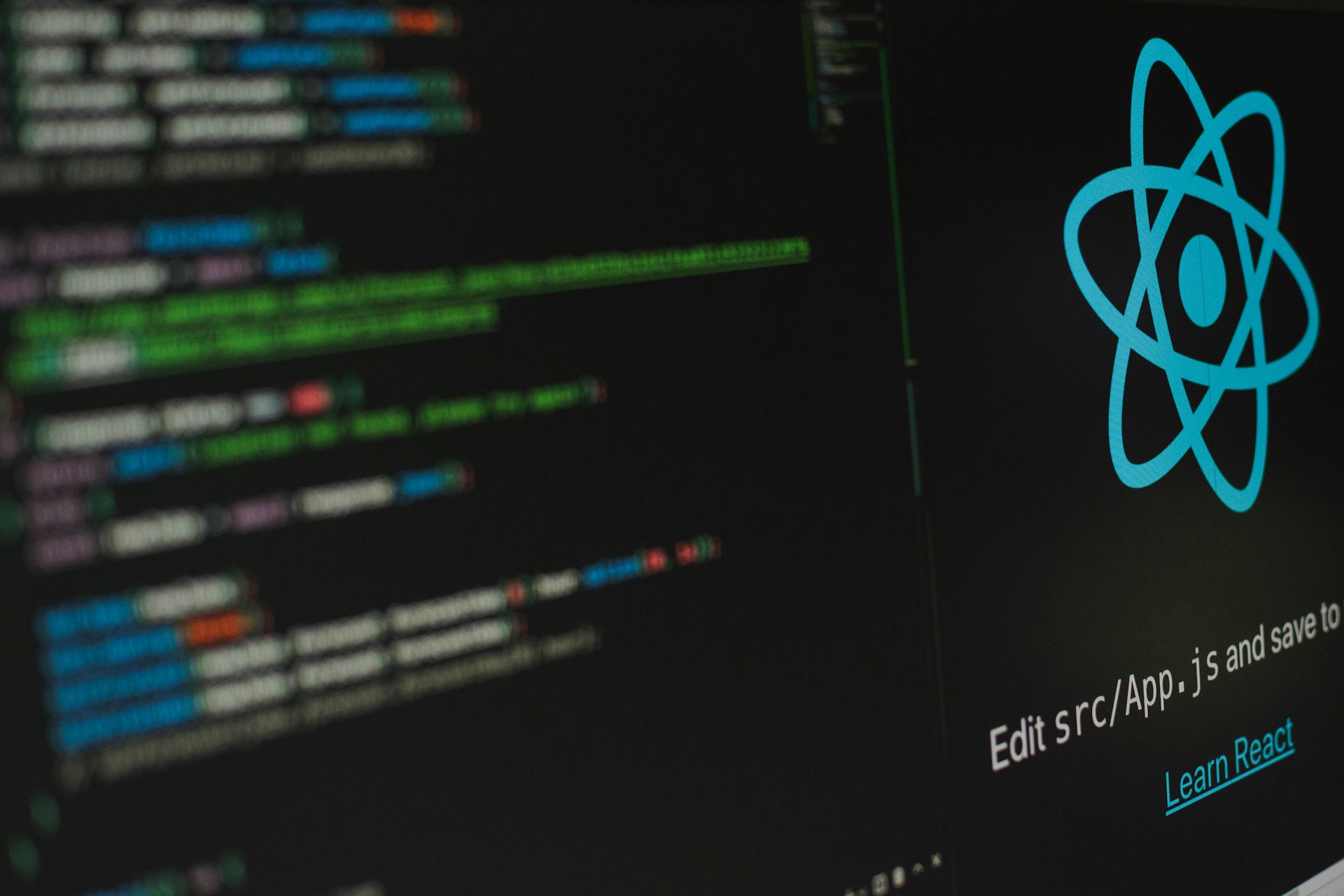
Laravel Sanctum Authentication in React Native App
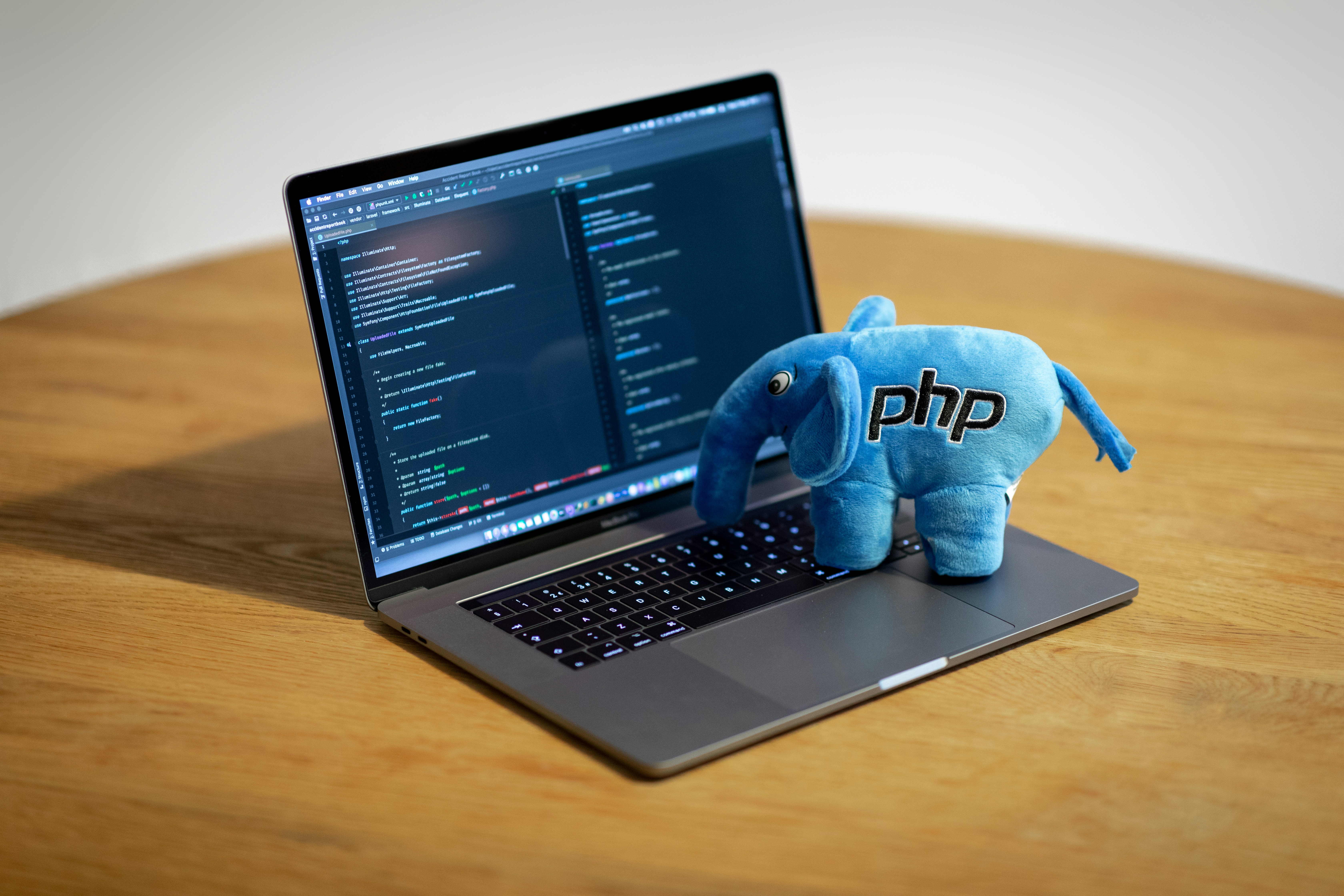